The stock market has gone through a pretty volatile period during the last two years. What seemed to be a stable growth of the worldwide stock market came to an abrupt halt in February 2020 when the corona pandemic took a toll on businesses all around the world.
This abrupt crash is also visible in the Dow Jones Industrial Average. The DJIA tracks 30 large and publicly-owned companies such as Apple, Cisco, Coca-Cola and many more. The graph below shows the price development of this index over the last two years. You can clearly see the big impact that the pandemic had on the stock prices of the companies that are included in the Dow Jones.
Price development of the Dow Jones Industrial Average
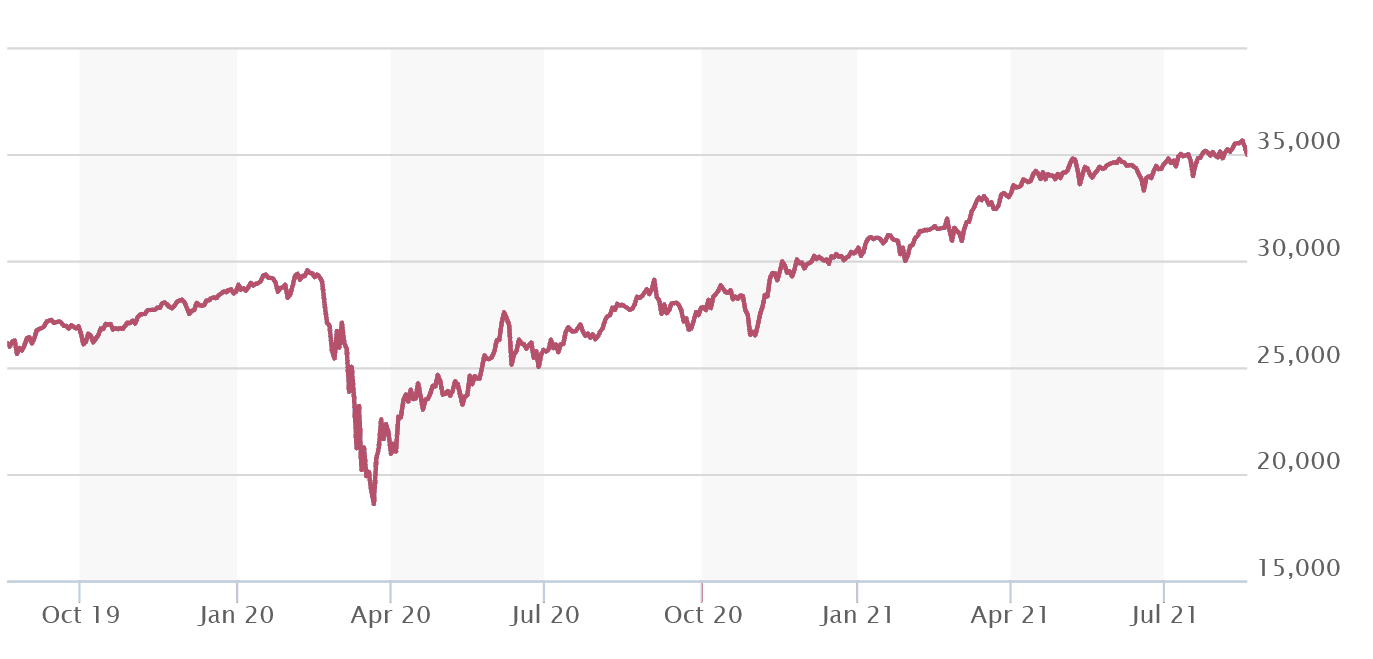
One particular thing that sparked my interest was how the different stocks in the DJIA recovered from the pandemic. Sadly, I was not able to find a finance website that is able to show the price development of all stocks in the DJIA at the same time.
The best website I could find was barchart.com, which is shown below. It allowed me to select up to five stocks and compare them visually, which was a good start but insufficient for my goal. All other websites that I found used similar restrictions that either limited the number of stocks or the timeframe that could be viewed.
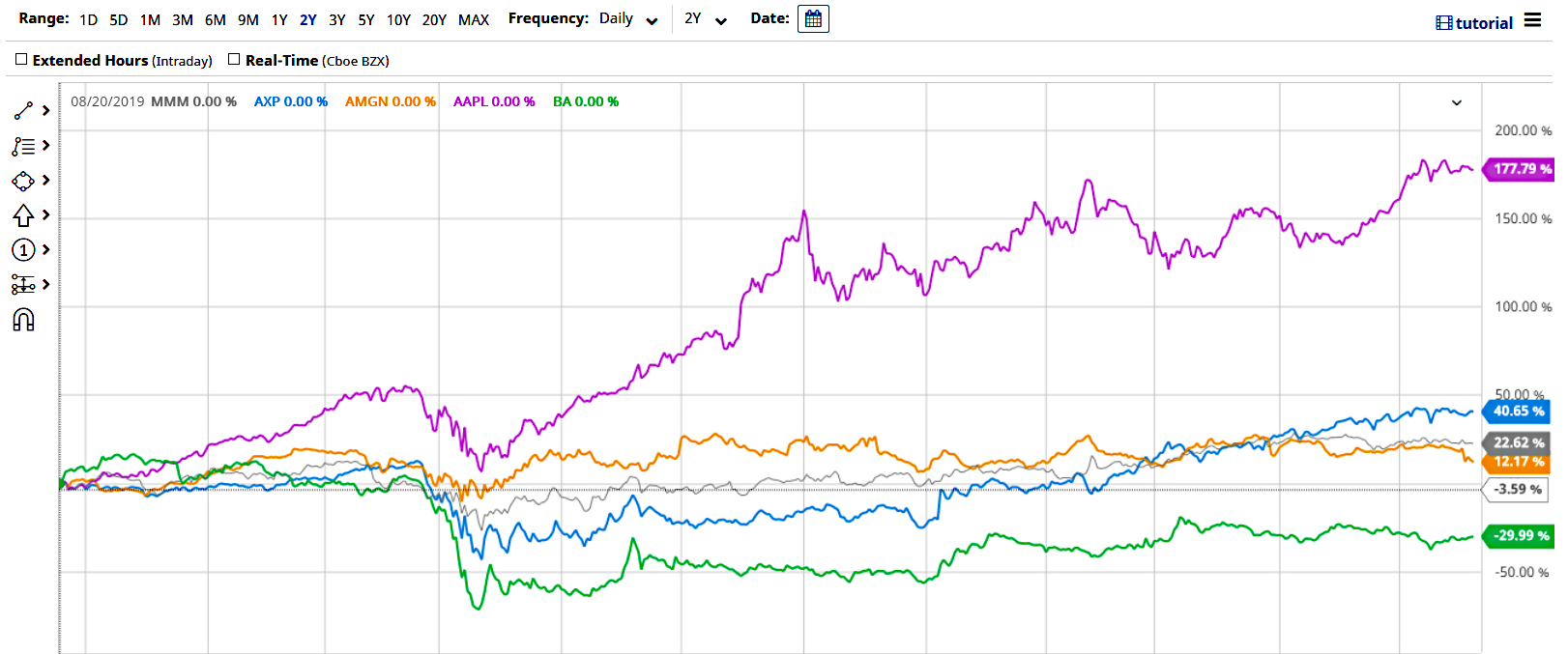
Because I could not find a website that fit my needs, I decided to create my own program that is capable of fetching and comparing stock prices. During the development of this program, I had two main goals in mind:
- Firstly, my program should be flexible regarding the number of stocks that it can process. The finance websites that I previously found only showed a limited number of stocks at a time. Therefore, my new program should be able to fetch stock data in bulk, merge it and return it as one big data frame.
- Secondly, I want to flexibly set a timeframe that should be fetched. This means that my program should have access to long-time historic stock data and be able to filter this data by date.
Programming the stock downloader
At first, I had to find a reliable data source. During my research, I found the Alphavantage REST API. This API provides historical stock data that reaches up to 20 years back in time. Furthermore, the stock prices are already adjusted and every user can issue five free requests per minute for free.
Using this API, I was able to program a python module that I called “stockdownloader”. This module reads in a list of stocks, an API key and a timeframe. The module will then split the list into multiple requests, download the stock data from Alphavantage, piece it together and return the result as one big data frame. The image below illustrates this process.

The downloader is available as a pip package, which can be installed with a single command. The usage of this package is also very easy. You simply pass a list of stocks, an API key and optionally a timeframe to the downloader. The stock downloader then automatically fetches all stocks in the list and gives back a data frame that contains the merged stock data.
The snippet below shows how to create a list of stocks, pass the list to the stock downloader and save the results in a CSV file. The full code example and its output can be viewed here.
import stockdownloader
#Define the stocks that should be downloaded
stocks = {
"MMM" :"3M",
"AXP" :"AmericanExpress"
}
#Pass the stocklist to the downloader
results = stockdownloader.downloadFromDict(stocks,apikey="key",startdate="2019-08-08",enddate="2021-08-08")
#Save the results to a csv file
results.to_csv("output.csv", index = False)
This code snippet will fetch the stock prices of the companies 3M and AmericanExpress from August 8, 2019 to August 8, 2021. I included a screenshot of the obtained data below. As you can see, the downloader fetches in-depth data about the two stocks like their opening prices, closing prices, daily highs, daily lows, volumes and dividends. All this information was merged by date to make comparing the stocks easy.

Comparing the recovery of stocks using the stock downloader
To solve my initial problem, I created a more sophisticated python script that passes all 30 stocks in the DJIA to the downloader and visualizes the results using Plotly. This code example is documented here. Using the stock downloader, I was able to easily generate the following interactive graph.
You can filter and explore the data yourself. A double click on a stock name in the legend will isolate its trace and a single click will toggle the visibility of the trace. A small example:
- Double click on “Boeing” and then add the company “Travelers” with a single click. Now you should only see the stock prices of the two travel companies. By looking at the graph, you can see that both stock prices took a big hit during the pandemic and are still struggling to recover.
- Now, also add the tech companies “Apple”, “Microsoft” and “Salesforce” with a single click. You will see that the tech companies recovered pretty quickly and are able to outperform the travel companies by a lot.
I will leave it up to you to pick the stocks that you are interested in and compare them yourself. I myself found it very interesting to go through the different stocks and compare their recession and recovery shapes.
Get the stock downloader
This article shows how my downloader can be used to flexibly download and analyze stock data. If you would like to experiment with the stock downloader and conduct your own analysis, feel free to download the python package below. The package contains the stock downloader, which can be installed with a single command. It furthermore includes a small documentation and code examples so you can start to download stock data right away.